Design and Analysis of Algorithm Lab 3 | Read Now
Design and Analysis of Algorithm Lab 3
3A] Write a Java Program to read two integers a and b. Computer a/b and print when b is not zero. Raise an exception when b is equal to zero
3B] Write a Java Program that implements a multi-thread application that has three threads. The first thread generates a random integer for every 1 second; the second thread computes the square of the number and prints; the third thread will print the value of the cube of the number.
3A] Program Code
import java.util.Scanner; public class lab3a { lab3a() { int a,b; float c; Scanner sc=new Scanner(System.in); try { System.out.println("Enter the value of a"); a=sc.nextInt(); System.out.println("Enter the value of b"); b=sc.nextInt(); sc.close(); if(b==0) throw new ArithmeticException(" Divide by Zero Error"); c=(float)a/b; System.out.println("value of a is: "+a); System.out.println("value of b is: "+b); System.out.println("a/b is: "+c); } catch(ArithmeticException e) { System.out.println("ERROR..!!"); e.printStackTrace(); } } public static void main(String srgs[]) { new lab3a(); } }
Output

3B] Program Code
import java.util.*; class second implements Runnable { public int x; public second (int x) { this.x=x; } public void run() { System.out.println("Second thread:Square of the number is "+x*x); } } class third implements Runnable { public int x; public third(int x) { this.x=x; } public void run() { System.out.println("third thread:Cube of the number is "+x*x*x+"\n"); } } class first extends Thread { public void run() { int num=0; Random r=new Random(); try { for(int i=0;i<5;i++) { num=r.nextInt(100); System.out.println("first thread generated number is "+num); Thread t2=new Thread (new second(num)); t2.start(); Thread t3=new Thread(new third(num)); t3.start(); Thread.sleep(1000); } } catch(Exception e) { System.out.println(e.getMessage()); } } } public class lab3b { public static void main(String args[]) { first a=new first(); a.start(); } }
Output
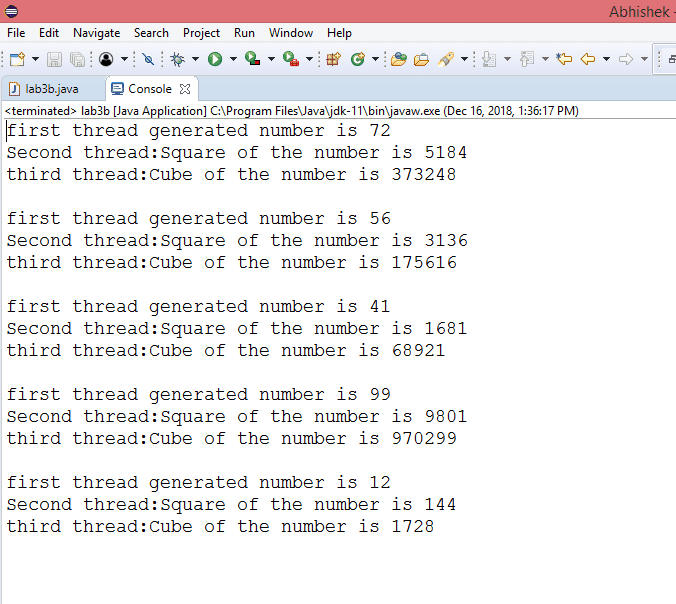